[ 작업 환경 ]
Windows10
jdk-15
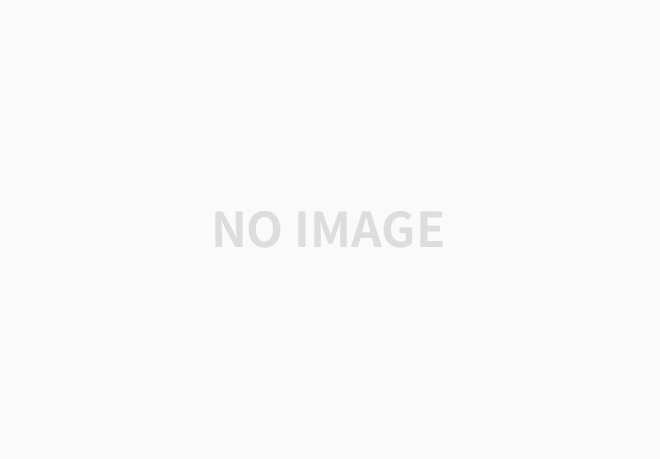
[ 문제 ]
• 첨부되는 Coin 클래스를 확장(extend)하여 UnfairCoin 클래스를 만들어 보시오. UnfairCoin 클래스는 앞면이 90% 확률로 나오고 뒷면이 10%로 나오는 coin을 나타내도록 하시오.
• CountFlips.java 를 수정하여 UnfairCoin이 90% 확률로 앞면이 나오는지 double 값 형태로 계산하여 나타내시오.
• 아래와 같이 cmd 입력창에서 결과를 확인할 수 있어야 한다.
java CountFlips
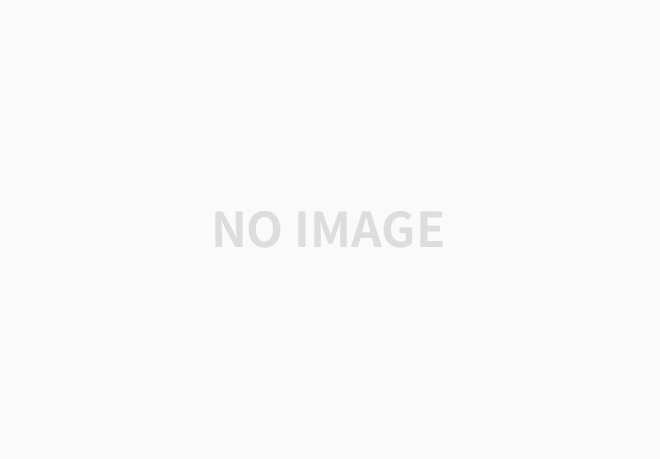
UnfairCoin.java_part 파일 참조
public class UnfairCoin extends Coin {
private double HeadProb;
public UnfairCoin(double inputHeadProb) {
//Add your logic
}
public void flip () {
//Add your logic
}
}
1. Coin.java
50% 확률로 앞, 뒷면이 나오는 코인 객체 예시
>>> 90% 확률로 앞이 나오는 코인 객체 만들기 전에 참고용으로만 보시면 됩니다.
//********************************************************************
// Coin.java Java Foundations
//
// Represents a coin with two sides that can be flipped.
//********************************************************************
public class Coin
{
protected final int HEADS = 0; // tails is 1
protected final int TAILS = 1; // tails is 1
protected int face; // current side showing
//-----------------------------------------------------------------
// Sets up this coin by flipping it initially.
//-----------------------------------------------------------------
public Coin ()
{
flip();
}
//-----------------------------------------------------------------
// Flips this coin by randomly choosing a face value.
//-----------------------------------------------------------------
public void flip ()
{
face = (int) (Math.random() * 2);
}
//-----------------------------------------------------------------
// Returns true if the current face of this coin is heads.
//-----------------------------------------------------------------
public boolean isHeads ()
{
return (face == HEADS);
}
//-----------------------------------------------------------------
// Returns the current face of this coin as a string.
//-----------------------------------------------------------------
public String toString()
{
return (face == HEADS) ? "Heads" : "Tails";
}
}
2. UnfairCoin.java 작성
public class UnfairCoin extends Coin {
private double HeadProb;
protected final int HEADS = 0; // tails is 1
public UnfairCoin(double inputHeadProb) {
//Add your logic
HeadProb = inputHeadProb;
flip();
}
public void flip () {
//Add your logic
face = (int) (Math.random() / HeadProb);
}
//-----------------------------------------------------------------
// Returns true if the current face of this coin is heads.
//-----------------------------------------------------------------
public boolean isHeads ()
{
return (face == HEADS);
}
//-----------------------------------------------------------------
// Returns the current face of this coin as a string.
//-----------------------------------------------------------------
public String toString()
{
return (face == HEADS) ? "Heads" : "Tails";
}
}
3. CountFlips.java 작성
앞면이 나올 확률을 입력받아 결과값을 출력합니다.
//********************************************************************
// CountFlips.java Java Foundations
//
// Demonstrates the use of a programmer-defined class.
//********************************************************************
import java.util.Scanner;
public class CountFlips
{
//-----------------------------------------------------------------
// Flips a coin multiple times and counts the number of heads
// and tails that result.
//-----------------------------------------------------------------
public static void main (String[] args)
{
final int FLIPS = 50000;
int heads = 0, tails = 0;
Scanner scan = new Scanner(System.in);
System.out.println("Enter the head probability:");
double headProb = scan.nextDouble();
UnfairCoin myCoin = new UnfairCoin((double)headProb);
for (int count=1; count <= FLIPS; count++)
{
myCoin.flip();
if (myCoin.isHeads())
heads++;
else
tails++;
}
System.out.println ("Number of flips: " + FLIPS);
System.out.println ("Number of heads: " + heads);
System.out.println ("Number of tails: " + tails);
}
}
4. 소스 실행 예시
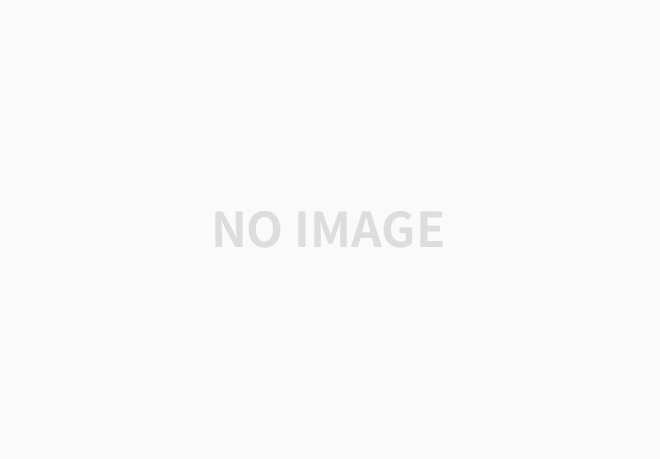